-----------------------------------------------
AOP stands for Aspect Oriented Programming. It simplifies the development of Application.
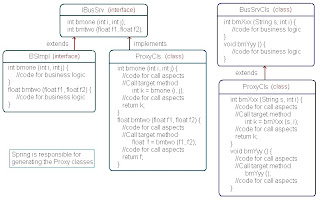
Provide the Service class –
------------------------------------------
package pack;
public interface IBusSrv {
int bmone (int I, int j);
float bmtwo (float f1, float f2);
}
package pack;
public class BSImpl implements IBusSrv {
public int bmone (int i, int j) {
System.out.println(“-----bmone()------“);
Return i+j;
}
public float nmtwo (float f1, float f2) {
System.out.println(“-------bmtwo()-------“);
return f1+f2;
}
}
package pack;
public class BusSrvCls {
public void bXxx(String s, int i) {
System.out.println(“--------bmXxx()---------“);
}
public void bmYyy() {
System.out.println(“----------bmYyy()------“);
}
}
Provide Aspect Classes –
-----------------------------------------
package pack;
import org.springframework.apo.MethodBeforeAdvice;
import org.springframework.apo.AfterReturningAdvice;
public class OurAspect implements MethodBeforeAdvice, AfterReturningAdvice {
public void before (Method met, Object[] args, Object target) throws Throwable {
System.out.println(“…….before…..”+met.getName());
System.out.println(“…….before…..”+args.length);
System.out.println(“…….before…..”+target.getClass());
}
public void afterReturning (Object rval, Method met, Object[] args, Object target)
throws Throwable {
System.out.println(“…….aR…..”+rval);
System.out.println(“…….aR…..”+met.getName());
System.out.println(“…….aR…..”+args.length);
System.out.println(“…….aR…..”+target.getClass());
}
}
Provide the info about the Service classes and Aspect classes in spring-servlet.xml file -
-----------------------------------------------------------------------------------
spring-servlet.xml file –
< bean name=”xxxTergate” class=”pack.BSImpl”>< /bean>
< bean name=”yyyTergate” class=”pack.BusSrvCls”>< /bean>
< bean name=”osBean” class=”pack.OurAspect”>< /bean>
< bean id=”xxx” class=”org.springframework.aop.framework.ProxyFactoryBean”>
< property name=”target”>
< ref bean=”xxxTarget”>< /ref>
< /property>
< property name=”interceptorNames”>
< list>
< value> osBean< /value>
< /list>
< /property>
< property name=”proxyInterfaces”>
< value> pack.IBusSrv < /value>
< /property>
< /bean>
< bean id=”yyy” class=”org.springframework.aop.framework.ProxyFactoryBean”>
< property name=”target”>
< ref bean=”yyyTarget”>< /ref>
< /property>
< property name=”interceptorNames”>
< list>
< value> osBean< /value>
< /list>
< /property>
< property name=”proxyTargetClass”>
< value> true< /value>
< /property>
< /bean>
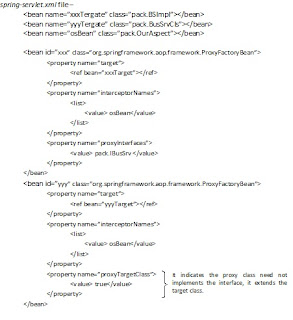
When the application calls getBena(“xxx”) method of spring container object a proxy object will be return. This object contains the code that used our aspect or advice and the target object based on BSImpl object.
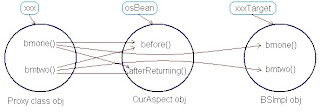
When bmone() method is invoked on the Proxy, it calls before() method, bmone() method on target object and finally it calls afterReturning() method. OurAspect acts as an intercepter.
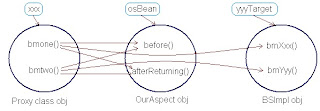
When the application calls getBean(“yyy”) method of the spring container object, a proxy object will be return and this object takes care of using OurAspect and BusServCls object.
Provide Main application Classes –
-----------------------------------------------------
package pack;
public class AOPTestClass {
public static void main (Sting [] args) throws Exception {
ApplicationContext sc = new ClassPathXmlApplicationContext
(“applicationContext.xml”);
Object obj;
Obj = sc.getBean(“xxx”);
IBusSrv ibs = (IBusSrv) obj;
ibs.bmone(10,20);
ibs.bmtwo(10.01f, 20.02f);
obj = sc.getBean(“yyy”);
BusSrvCls bsc = (BusSrvCls) obj;
bsc.bmXxx(“aa”, 10);
bsc.bmYyy();
}
}
Crosscutting concern –
-------------------------------
The code to manage the transactions and the code to manage the security is required at multiple parts and hence these are called as Crosscutting concern.
Disadvantage of OOPs –
-------------------------------
OOPs allows us to reuse the code by creating subclasses and subclasses, but creating the sense the super classes does not make sense in some classes (creating a Person class as a sub class of Dog class, creating TransactionManager as subclass of SecurityMamager does not make sense)
AOP solves OOPs problem –
-------------------------------
Aspect Oriented Programming solves this problem. It allows us to reuse the code. An aspect is a crosscutting concern. Login, Security management, Transaction management are the good example of aspect.
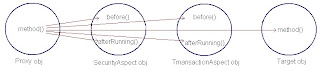
Join-Point –
-------------------------------
Using an aspect is called as advising. The point at which the aspect is advice is called as join-point. Before calling a method and after calling a method are examples of joint-points.
The code of OurAdvice is used along with BSImpl and BusSrvCls that is we have reused the code of OurAspect in multiple classes. Spring supports the transaction management by using aspect oriented programming.
No comments:
Post a Comment